A simple example of adding a document to the Firestore:
from google.cloud import firestore
#Make a note in the firestore
firestore_client = firestore.Client()
doc_ref = firestore_client.collection(u'CollectionsNameHere').add({
u'propertyone': u'thisisavalue',
u'propertytwo': 2,
})
logging.warning(doc_ref[1].id)
.add returns a tuple of the date that the document was written into the firestore, and a document reference to the written document. .add lets Firestore create a document ID to the written document: to figure out what the ID is, the last line accesses the tuple and pulls out the document ID.
An item that bit me: the official documentation for Firestore states that .add and .doc().set() are equivalent. That’s true, but the returns from both of those functions are different.
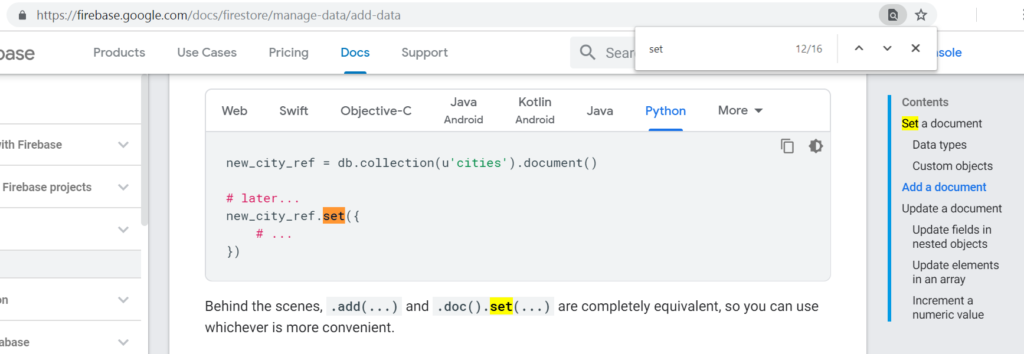
.add returns a tuple of the document creation time and then a reference to the added document. However, .set returns a WriteResult, not a tuple. The WriteResult contains the time the document was updated/set in the property update_time. Make sure to use the correct function as necessary.
Set Documentation
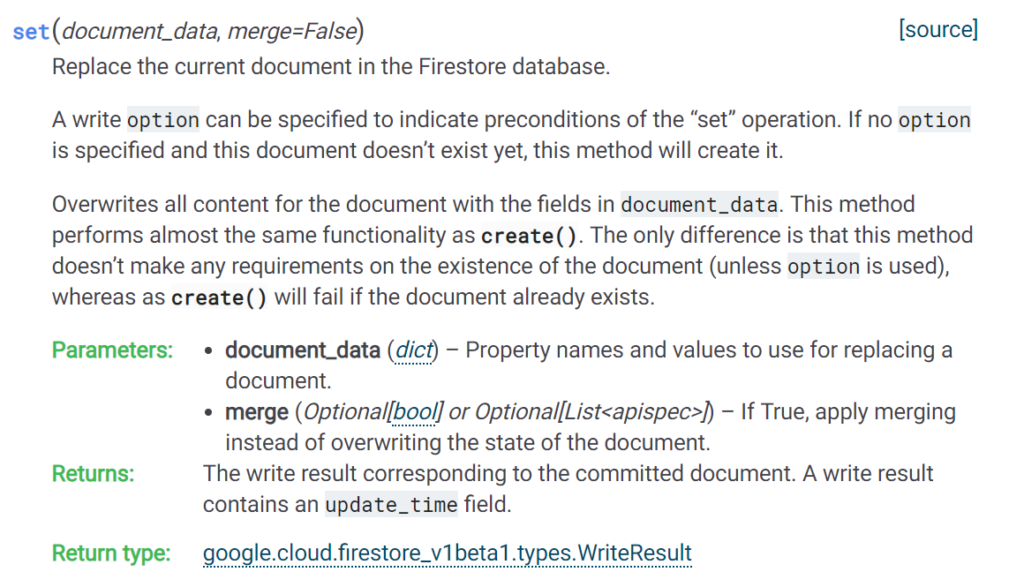
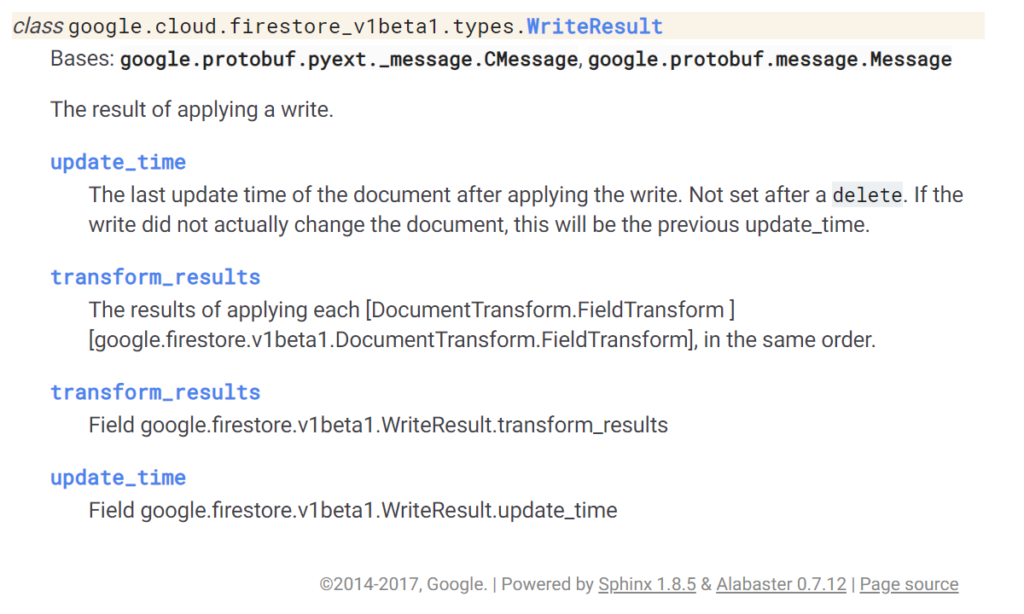
Add Documentation
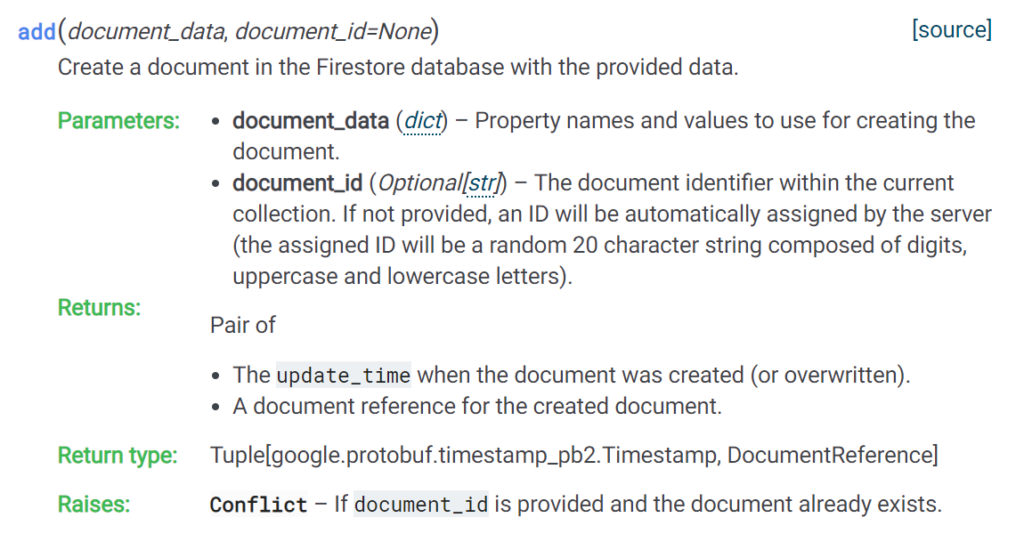